Blogspot - vijayinterviewquestions.blogspot.in - Interview Questions
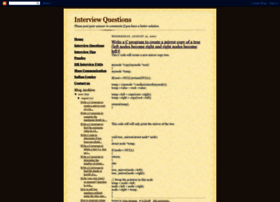
General Information:
Latest News:
Write a C program to create a mirror copy of a tree (left nodes become right and right nodes become left)! 15 Aug 2007 | 11:28 pm
This C code will create a new mirror copy tree. mynode *copy(mynode *root) { mynode *temp; if(root==NULL)return(NULL); temp = (mynode *) malloc(sizeof(mynode)); temp->value = root->value; temp->le...
Write a C program to compute the maximum depth in a tree? 15 Aug 2007 | 11:27 pm
int maxDepth(struct node* node) { if (node==NULL) { return(0); } else { int leftDepth = maxDepth(node->left); int rightDepth = maxDepth(node->right); if (leftDepth > rightDepth) return(leftDepth+1); e...
Write a C program to find the mininum value in a binary search tree. 15 Aug 2007 | 11:26 pm
Here is some sample C code. The idea is to keep on moving till you hit the left most node in the tree int minValue(struct node* node) { struct node* current = node; while (current->left != NULL) { c...
Write C code to determine if two trees are identical . 15 Aug 2007 | 11:24 pm
Here is a C program using recursion int identical(struct node* a, struct node* b) { if (a==NULL && b==NULL){return(true);} else if (a!=NULL && b!=NULL) { return(a->data == b->data && identical(a->lef...
Write a C program to delete a tree (i.e, free up its nodes) 15 Aug 2007 | 11:23 pm
Free up the nodes using Postorder traversal!.
Write a C program to determine the number of elements (or size) in a tree. 15 Aug 2007 | 11:21 pm
int tree_size(struct node* node) { if (node==NULL) { return(0); } else { return(tree_size(node->left) + tree_size(node->right) + 1); } }
Write a C program to find the depth or height of a tree. 15 Aug 2007 | 11:13 pm
Here is some C code to get the height of the three tree_height(mynode *p) { if(p==NULL)return(0); if(p->left){h1=tree_height(p->left);} if(p=>right){h2=tree_height(p->right);} return(max(h1,h2)+1); }...
Write your own trim() or squeeze() function to remove the spaces from a string. 15 Aug 2007 | 11:08 pm
#include char *trim(char *s); int main(int argc, char *argv[]) { char str1[]=" Hello I am Good "; printf("\n\nBefore trimming : [%s]", str1); printf("\n\nAfter trimming : [%s]", trim(str1)); getch(...
How to add two numbers without using the plus operator? 15 Aug 2007 | 11:05 pm
Actually, SUM = A XOR B CARRY = A AND B On a wicked note, you can add two numbers wihtout using the + operator as follows a - (- b)
How to generate prime numbers? How to generate the next prime after a given prime? 15 Aug 2007 | 11:03 pm
This is a very vast subject. There are numerous methods to generate primes or to find out if a given number is a prime number or not. Here are a few of them. I strongly recommend you to search on the ...